Widget Installation and Settings
Description
The VerifiedEmail widget is an easily customizable email verification widget. It supports as-you-type verification or verification upon form submission, and also offers custom verification on demand. With flexible settings, the widget includes support for events and public methods. It can also be integrated with jQuery.
Creating A Widget
Before adding a widget to your web page, you need to create the widget in your VerifiedEmail account.
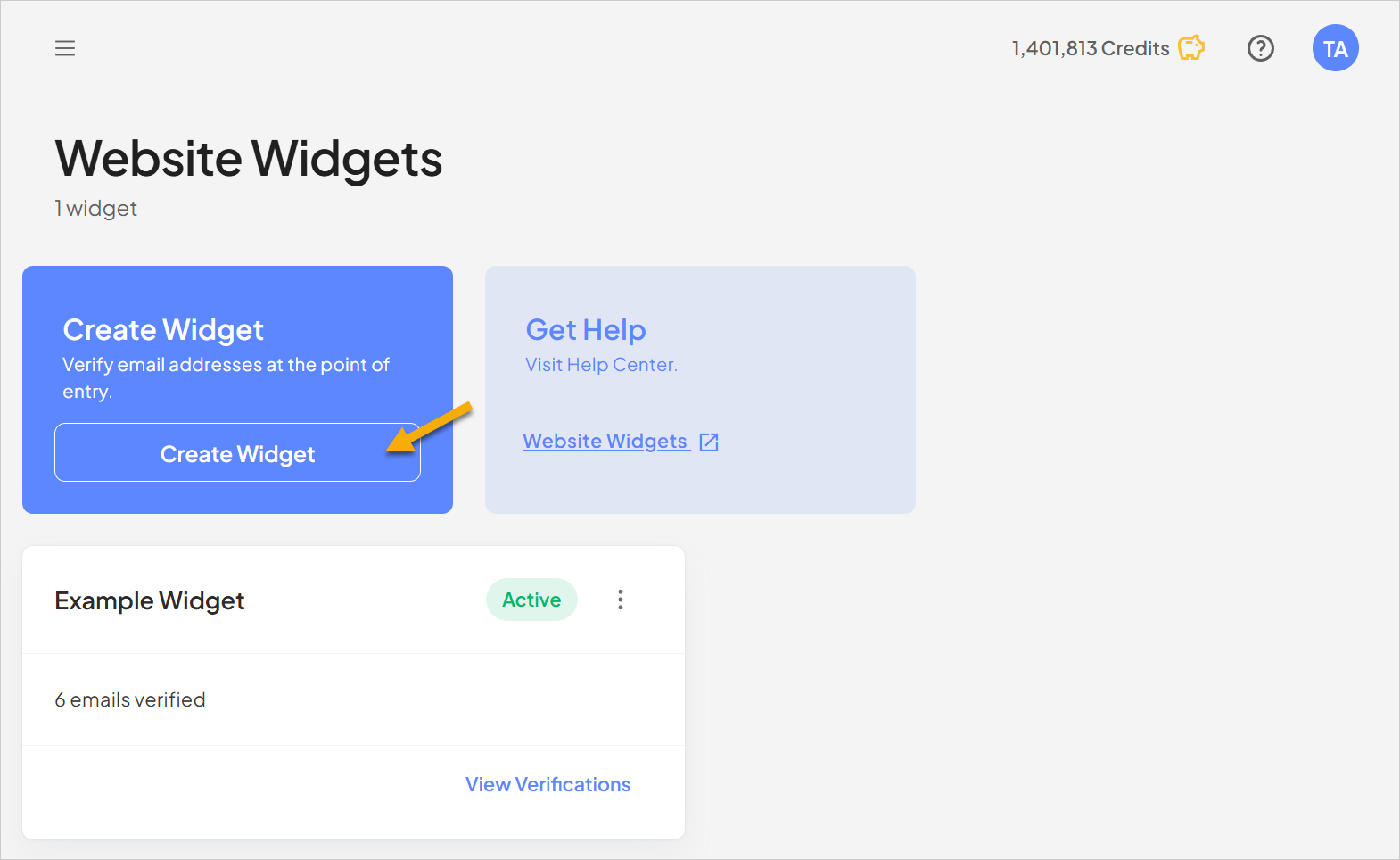
For more information, please see Website Widgets.
Adding a Widget to a Web Page
In VerifiedEmail, click the triple dot button of the widget you want to add to your web page, and choose View Embed Code.
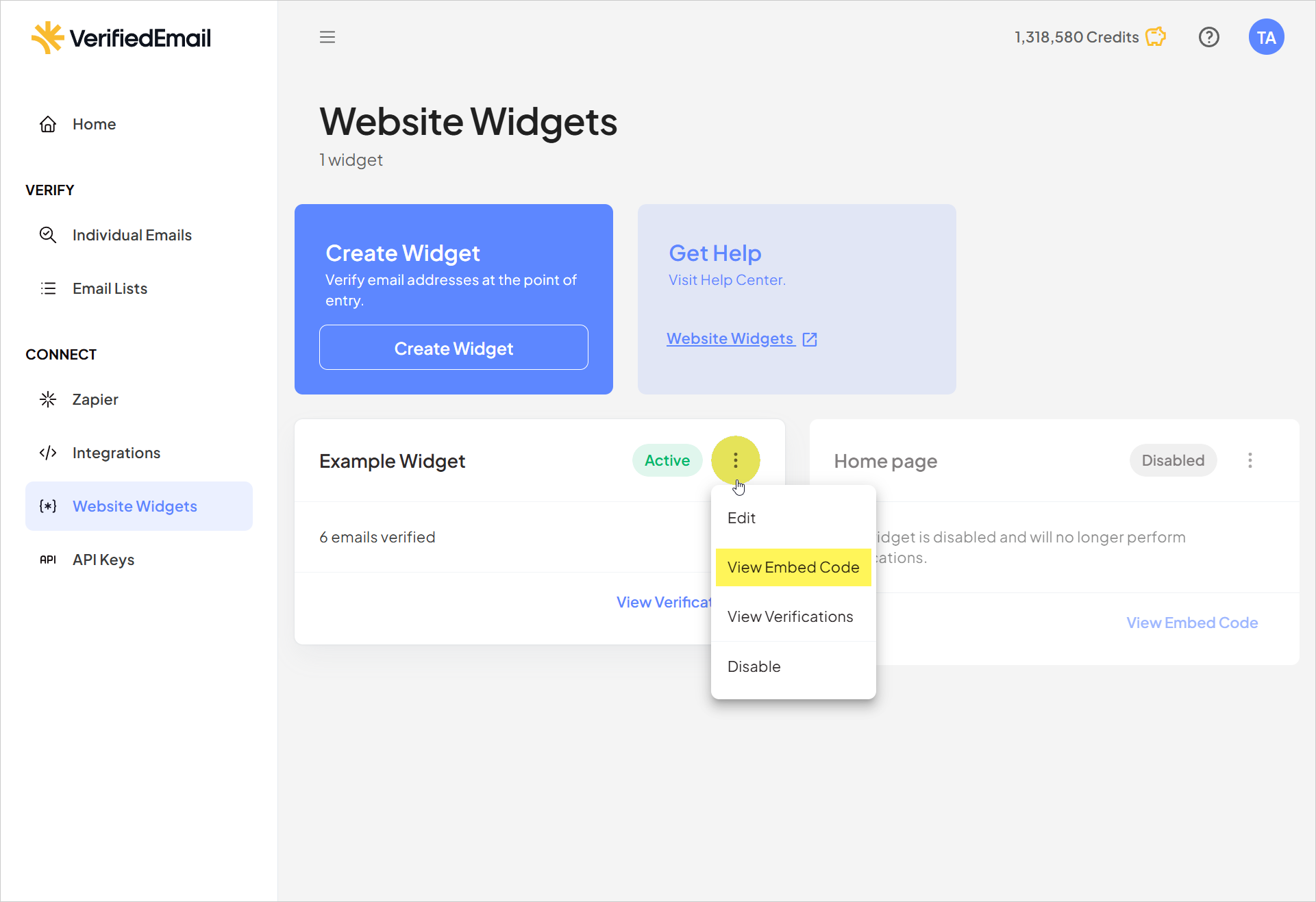
Option 1: Simple Approach
Copy and paste the script from the Simple tab of the Embed Widget dialog in VerifiedEmail into the <head> of your page. For example:
src="https://app.verified.email/widget/verified.email.min.js?token=wgt_fk2e7midpx9ypyhd50yxfys87hfbcts6&recaptchaSiteKey=verified-email-435515" >
</script>
Add data-verified-email="verified" to a field used to collect an email address.
- If the field is located inside the <form>, VerifiedEmail will automatically verify the email address before the form can be submitted.
- If the field is not located inside the <form> please refer to the Advanced option, below.
That's it! When your visitor enters their email address into the field and submits the form, VerifiedEmail will perform email validation and, if their email address is valid, submit the form. Otherwise, an error will be shown to the visitor.
VerifiedEmail supports additional configuration options and callbacks which can be provided as data-* variables of the email field.
Option 2: Advanced Approach
Copy and paste the script from the Advanced tab of the Embed Widget dialog in VerifiedEmail into the <head> of your page. For example:
src="https://app.verified.email/widget/verified.email.min.js?token=wgt_fk2e7midpx9ypyhd50yxfys87hfbcts6&recaptchaSiteKey=verified-email-435515" ></script>
Create an initialization function to initialize a widget instance by passing a field element or selector and configuration options.
function init() {
verifiedEmail.initialize('#email-field', { // Email field element or selector.
timeout: 30, // Optional. Default: 30
submit: true, // Optional. Default: true
live: false, // Optional. Default: false
validStatuses: ["deliverable", "full"], // Optional. Default: ["deliverable", "full"]
messages: {
// Optional. Override default validation status messages. For more information, see Settings, below.
},
callbacks: {
// Optional. Refer to Events, below, for all available callbacks
}
});
}
</script>
Call the init() function on page load or based on your integration needs.
That's it! Now the widget will be initialized and attached to the field you provided. Verifications will work based on your configuration.
For more options, please see all available configuration options, below.
Option 3: Headless Approach
VerifiedEmail offers a JavaScript API, allowing you to perform verifications programmatically rather than attaching a widget to a field.
Copy and paste the VerifiedEmail script from the Headless tab of the Embed Widget dialog into the <head> of your page. For example:
src="https://app.verified.email/widget/verified.email.min.js?token=wgt_fk2e7midpx9ypyhd50yxfys87hfbcts6&recaptchaSiteKey=verified-email-435515" >
</script>
Initialize a widget instance without passing a field element or selector.
document.addEventListener('ve.initialize', () => {
const instanceId = verifiedEmail.initialize(null, { // Do not pass email field element or selector.
timeout: 30, // Optional. Default: 30
validStatuses: ["deliverable", "full"], // Optional. Default: ["deliverable", "full"]
messages: {
// Optional. Override default validation status messages. For more information, see Settings, below.
},
callbacks: {
// Optional. Refer to Events, below, for all available callbacks
}
});
});
</script>
Call the VerifiedEmail JavaScript API directly when needed.
verifiedEmail.verify("visitor.email@gmail.com", instanceId)
.then( result => {
// Your code. result" will contain a verification response from the server.
})
.catch( error => {
// error will contain a string describing an error
})
</script>
That's it!
For more options, please see all available configuration options, below.
Connect Features
Regardless of whether you implement the Simple, Advanced, or Headless approach, the widget offers the following features:
⚡ You can use the global variable verifiedEmail for getting access to the widget.
⚡ Create multiple separate widget instances for each email input.
⚡ New instances can be created with the method verifiedEmail.initialize(<selector or field>, <settings object>).
⚡ To get access to already existing instances, use array verifiedEmail.instances[].
⚡ You can use the method .verify() on the field for the verification on demand. This method is available on the DOM node and also in case you are using jQuery, use one of these $(selector).verify() / jQuery(selector).verify()
⚡ Please use the method verifiedEmail.verify('example@mail.com').then((verificationObject) => {}).catch() for email verification on demand.
⚡ We can pass config options with an object or via html attributes on the field.
⚡ You can use a selector for the input field or a variable with it.
Verification Object
After the verification process, we pass a verification email object. For complete details, please see Verification Results.
Settings
In all configurations, it is possible to use the following options:
Option | Data-Attr | Defaults | Type | Description |
---|---|---|---|---|
‘token’ | string | Your token, please see documentation for more information. | ||
‘selector’ | string | Input selector | ||
‘submit’ | verify-submit="true" | false | bool | Use submit form event for starting the email validation process |
‘live’ | verify-live="true" | false | bool | Enable live validation Important: Enabling the live option can potentially result in multiple validation queries–and consumption of multiple credits– per entry. For example, if a user enters a partial email address (e.g., john@something.co) and stops typing, we make a validation request. If the user resumes typing after that (e.g., adding an “m” to the .com registry), we make an additional validation request, so an additional credit will be consumed. |
‘debounceDelay’ | verify-delay="700" | 700 | Only available when “live” is set to true | |
‘timeout’ | verify-timeout | 30(s) | Unsigned integer | Timeout setting response from our service, by default it set as 30 seconds. |
‘valid’ | verify-valid | deliverable, full | List of statuses, separated with "," | List of email statuses for success validation |
‘messages’ | verify-<status_code> | string | Message for the live verification. If we initialize it with a config, we can pass the field messages with an object where the key is email status code. If we use html attributes, we can pass attribute verify-<status_code> on the input field | |
‘callbacks’ | Use the following: - onBeforeValidation - onLocalValidation - onRequest - onResponse - onSuccess | event | Callback events |
Events
Events should be passed through the parameter callbacks in the settings or html attributes.
The validation process has the following steps:
- Validate email address on local machine.
- Send a request to our service to start the validation process.
- Receive the response of the success validation.
In addition to the standard options, you now have the access to the following events:
Option | Native Event Name | Events Parameters (Use event.details to receive) | Description |
---|---|---|---|
‘onBeforeValidation’ | ve.before.validation | email - string; instanceId: string; | Event before local string validation for email format |
‘onLocalValidation’ | ve.local.validation | email - string; instanceId: string; isValid: bool; | End of the email validation process |
‘onRequest’ | ve.request | email - string; instanceId: string; | Dispatch before the request to our service to get the verify object |
‘onResponse’ | ve.response | verifyObject - email verification object; instanceId: string; | The event dispatching after receiving the verification object from our service |
‘onSuccess’ | ve.success | verifyObject - email verification object; instanceId: string; | The callback is executed in case the email status exists in the list of success |
Additionally, you can add a custom event listener:
document.querySelector('#exampleID').addEventListener('ve.local.validation',(event) => {
const {email, isValid, instanceId} = event.detail;
// ..
})
'